Introduction to ChatGPT for Coding
ChatGPT has emerged as a powerful tool in the world of software development, offering developers new ways to approach coding tasks and problem-solving. This section explores the role of ChatGPT in coding and how it can enhance coding efficiency.
Understanding ChatGPT‘s Role in Coding
ChatGPT serves as an AI-powered coding assistant, capable of generating code snippets, explaining programming concepts, and helping developers troubleshoot issues. Its primary functions in the coding process include:
- Code Generation: ChatGPT can produce code snippets based on natural language descriptions of desired functionality. For example:
# Original code
def process_data(data):
result = []
for item in data:
if item % 2 == 0:
result.append(item * 2)
return result
# ChatGPT suggestion for refactoring
def process_data_refactored(data):
return [item * 2 for item in data if item % 2 == 0]
- Code Explanation: It can break down complex code segments and explain their functionality, making it easier for developers to understand unfamiliar code or learn new programming concepts.
- Debugging Assistance: By analyzing code snippets and error messages, ChatGPT can suggest potential fixes or identify issues in the code.
- Language Translation: It can help translate code from one programming language to another, facilitating cross-language development.
- Documentation Generation: ChatGPT can assist in creating code documentation, including function descriptions and usage examples.
While ChatGPT offers these capabilities, it’s important to note that it’s not a replacement for human programmers. Instead, it serves as a complementary tool to augment developer productivity and problem-solving abilities.
Enhancing Coding Efficiency with ChatGPT
Leveraging ChatGPT effectively can significantly boost coding efficiency in several ways:
- Rapid Prototyping: ChatGPT can quickly generate initial code structures or prototypes, allowing developers to iterate on ideas faster.
- Learning Aid: For developers learning new languages or frameworks, ChatGPT can provide explanations, examples, and best practices, accelerating the learning process.
- Code Refactoring: By suggesting alternative implementations or optimizations, ChatGPT can help improve code quality and performance.
- Problem-Solving Support: When faced with challenging coding problems, developers can use ChatGPT to brainstorm solutions or get hints on potential approaches.
- Time-Saving on Routine Tasks: For repetitive coding tasks, such as writing boilerplate code or simple utility functions, ChatGPT can save time by generating these quickly.
Here’s an example of how ChatGPT can assist in refactoring code:
# Original code
def process_data(data):
result = []
for item in data:
if item % 2 == 0:
result.append(item * 2)
return result
# ChatGPT suggestion for refactoring
def process_data_refactored(data):
return [item * 2 for item in data if item % 2 == 0]
In this case, ChatGPT suggested a more concise and Pythonic way to write the function using a list comprehension.
While ChatGPT can significantly enhance coding efficiency, it’s crucial to use it judiciously. Developers should always review and test the code generated by ChatGPT, as it may not always produce perfect or optimal solutions. Additionally, relying too heavily on AI-generated code can potentially hinder a developer’s growth and understanding of fundamental programming concepts.
By understanding ChatGPT’s role in coding and leveraging its capabilities effectively, developers can strike a balance between AI assistance and human expertise, ultimately leading to more efficient and productive coding practices.
Mistakes to Avoid When Using ChatGPT for Coding
While ChatGPT can be a powerful tool for coding assistance, it’s crucial to be aware of potential pitfalls. By understanding these common mistakes, you can maximize the benefits of AI-assisted coding while minimizing risks.
1. Overreliance on ChatGPT
One of the most significant mistakes developers make is becoming overly dependent on ChatGPT for their coding tasks. While the AI can provide quick solutions and code snippets, it’s essential to maintain your critical thinking and problem-solving skills.
2. Neglecting to Verify and Test Code
ChatGPT can generate code that appears functional at first glance but may contain errors or inefficiencies. Always verify and test the code thoroughly before implementation. For example:
# ChatGPT-generated code
def calculate_average(numbers):
return sum(numbers) / len(numbers)
# Potential issue: Division by zero if the list is empty
# Improved version
def calculate_average(numbers):
return sum(numbers) / len(numbers) if numbers else 0
3. Stunting Personal Growth
Relying too heavily on AI for coding solutions can hinder your learning process. Instead of immediately turning to ChatGPT, try to solve problems on your own first. Use the AI as a supplement to your knowledge, not a replacement for it.
4. Ignoring Project-Specific Requirements
ChatGPT may not always consider the unique constraints or requirements of your project. It’s crucial to provide context and ensure that the generated code aligns with your specific needs.
5. Misinterpreting ChatGPT Outputs
Another common mistake is misunderstanding or misusing the information provided by ChatGPT. This can lead to various issues in your coding process.
6. Assuming Perfect Accuracy
ChatGPT’s responses are based on its training data, which may not always be up-to-date or entirely accurate. For instance, it might suggest using deprecated functions or outdated libraries. Always cross-reference its suggestions with official documentation.
# ChatGPT might suggest:
import urllib2 # Deprecated in Python 3
# Instead, use:
import urllib.request # Python 3 compatible
7. Overlooking Context and Limitations
ChatGPT may not have access to your entire codebase or project structure. It’s important to adapt its suggestions to fit your specific context. Additionally, be aware that ChatGPT’s knowledge cutoff date means it may not be familiar with the latest programming languages, frameworks, or best practices.
8. Misunderstanding Complex Algorithms
For complex algorithms or data structures, ChatGPT might provide a simplified explanation that doesn’t capture all the nuances. It’s crucial to dig deeper and understand the underlying principles, especially for performance-critical code.
# ChatGPT might suggest a simple sorting algorithm:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# However, for large datasets, you might need a more efficient algorithm:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
By being aware of these potential mistakes, you can use ChatGPT more effectively in your coding process. Remember to view it as a tool to enhance your coding skills rather than a substitute for your own knowledge and judgment. Always verify, test, and understand the code you’re implementing, regardless of its source.
Best Practices for Using ChatGPT in Coding
While ChatGPT can be a powerful tool for coding assistance, it’s essential to use it wisely. This section outlines best practices to maximize the benefits of ChatGPT in your coding workflow while minimizing potential pitfalls.
Effective Code Generation
When using ChatGPT for code generation, follow these guidelines to ensure the best results:
- Be specific in your prompts: Provide clear, detailed instructions about what you want to achieve. Include information about the programming language, framework, and any specific requirements or constraints.
Example prompt: "Write a Python function that takes a list of integers as input, removes duplicates, and returns the sorted list in descending order. Use list comprehension if possible."
- Break down complex tasks: Instead of asking for an entire program at once, divide it into smaller, manageable components. This approach helps ChatGPT focus on specific functionalities and reduces the likelihood of errors.
- Review and test the generated code: Always thoroughly review and test the code provided by ChatGPT. Don’t assume it’s error-free or optimized. Run it through your development environment and conduct proper testing.
- Use ChatGPT for inspiration and learning: Treat the generated code as a starting point or learning resource rather than a final solution. Analyze the code, understand its logic, and modify it to fit your specific needs.
- Iterate and refine: If the initial output isn’t satisfactory, refine your prompt or ask follow-up questions. ChatGPT can often provide alternative solutions or explanations.
- Combine with other resources: Use ChatGPT in conjunction with official documentation, Stack Overflow, and other programming resources for a well-rounded approach to problem-solving.
Security Considerations
When using ChatGPT for coding tasks, keep these security considerations in mind:
- Avoid sharing sensitive information: Never include API keys, passwords, or other sensitive data in your prompts. ChatGPT doesn’t have access to your local environment, so it can’t retrieve or use this information securely.
- Validate security-related code: If you’re using ChatGPT to generate code related to authentication, encryption, or other security-sensitive areas, have it reviewed by a security expert or cross-reference with trusted security guidelines.
- Be cautious with generated SQL queries: If ChatGPT provides SQL queries, always validate them to prevent SQL injection vulnerabilities. Use parameterized queries and proper input sanitization.
- Check for outdated practices: ChatGPT’s knowledge cutoff means it might suggest outdated security practices. Always verify security recommendations against current best practices and standards.
- Use code analysis tools: Run generated code through static analysis tools to catch potential security issues that might not be immediately apparent.
- Understand the code you use: Don’t blindly copy-paste code from ChatGPT into your project. Ensure you understand how it works and its potential implications for your system’s security.
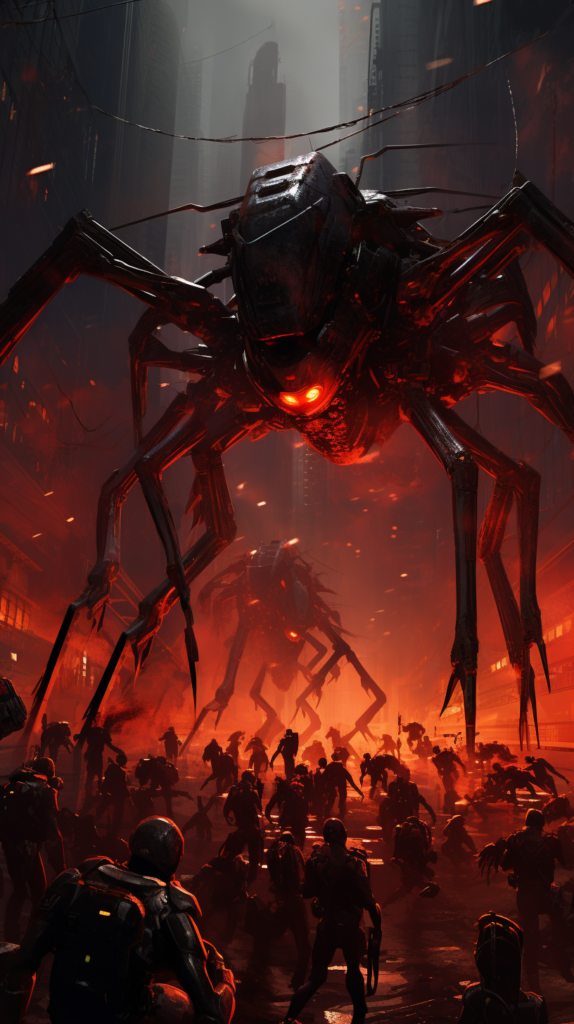
By following these best practices, you can harness the power of ChatGPT to enhance your coding process while maintaining code quality and security. Remember, ChatGPT is a tool to augment your skills and knowledge, not replace them. Your expertise and judgment as a developer remain crucial in creating robust, secure, and efficient code.
Do you want to know how ChatGPT compares with the new DeepSeek. Find out here in this article: DeepSeek vs ChatGPT: The Ultimate Showdown of Cutting-Edge AI Tools